A non-empty array A consisting of N integers is given. Array A represents numbers on a tape.
Any integer P, such that 0 < P < N, splits this tape into two non-empty parts: A[0], A[1], ..., A[P − 1] and A[P], A[P + 1], ..., A[N − 1].
The difference between the two parts is the value of: |(A[0] + A[1] + ... + A[P − 1]) − (A[P] + A[P + 1] + ... + A[N − 1])|
In other words, it is the absolute difference between the sum of the first part and the sum of the second part.
For example, consider array A such that:
A[0] = 3 A[1] = 1 A[2] = 2 A[3] = 4 A[4] = 3
We can split this tape in four places:
- P = 1, difference = |3 − 10| = 7
- P = 2, difference = |4 − 9| = 5
- P = 3, difference = |6 − 7| = 1
- P = 4, difference = |10 − 3| = 7
Write a function:
def solution(A)
that, given a non-empty array A of N integers, returns the minimal difference that can be achieved.
For example, given:
A[0] = 3 A[1] = 1 A[2] = 2 A[3] = 4 A[4] = 3
the function should return 1, as explained above.
Write an efficient algorithm for the following assumptions:
- N is an integer within the range [2..100,000];
- each element of array A is an integer within the range [−1,000..1,000].
Copyright 2009–2022 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
# you can write to stdout for debugging purposes, e.g.
# print("this is a debug message")
def solution(A):
if len(A) == 2:
return abs(A[0] - A[1])
arr = []
tmp1 = 0
tmp2 = sum(A)
for i in range(len(A)-1):
tmp1 = tmp1 + A[i]
tmp2 = tmp2 - A[i]
arr.append(abs(tmp1 - tmp2))
return min(arr)
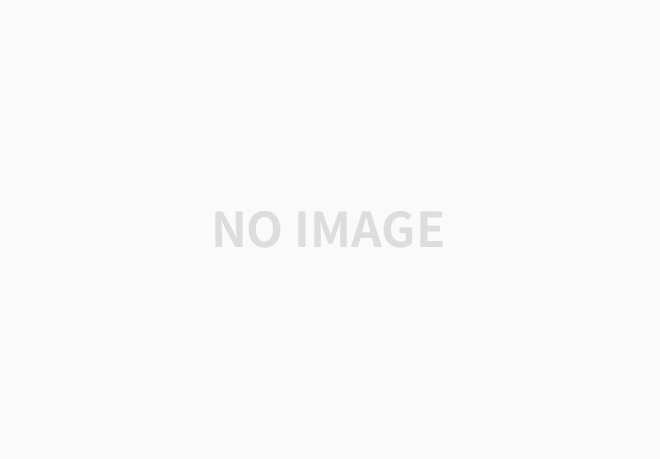
쓰잘데기 없이 멋있어 보이게 짠다고 내장함수 남발하면 time out의 칼날이 날아온다는 점 잊지 말자........
'Algorithm > Algorithm__Codility-Python' 카테고리의 다른 글
Lesson3 - PermMissingElem (0) | 2022.06.21 |
---|---|
Lesson3- FrogJmp (0) | 2022.06.21 |
Lesson2 - OddOccurrencesInArray (0) | 2022.06.21 |
Lesson2 - CyclicRotation (0) | 2022.06.21 |
Lesson1 - Binary Gap (0) | 2022.06.21 |